 |
|
|
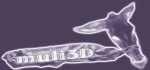 |
Reference: Muli3D: CMuli3DTexture Class Reference
CMuli3DTexture Class Reference#include <m3dcore_texture.h>
Inheritance diagram for CMuli3DTexture:
List of all members.
Detailed Description
CMuli3DTexture implements a standard 2-dimensional texture.
|
Public Member Functions |
result | Clear (uint32 i_iMipLevel, const vector4 &i_vColor, const m3drect *i_pRect) |
m3dformat | fmtGetFormat () |
result | GenerateMipSubLevels (uint32 i_iSrcLevel) |
uint32 | iGetFormatFloats () |
uint32 | iGetHeight (uint32 i_iMipLevel=0) |
uint32 | iGetMipLevels () |
uint32 | iGetWidth (uint32 i_iMipLevel=0) |
result | LockRect (uint32 i_iMipLevel, void **o_ppData, const m3drect *i_pRect) |
CMuli3DSurface * | pGetMipLevel (uint32 i_iMipLevel) |
result | UnlockRect (uint32 i_iMipLevel) |
Protected Member Functions |
| CMuli3DTexture (class CMuli3DDevice *i_pParent) |
result | Create (uint32 i_iWidth, uint32 i_iHeight, uint32 i_iMipLevels, m3dformat i_fmtFormat) |
m3dtexsampleinput | eGetTexSampleInput () |
result | SampleTexture (vector4 &o_vColor, float32 i_fU, float32 i_fV, float32 i_fW, const vector4 *i_pXGradient, const vector4 *i_pYGradient, const uint32 *i_pSamplerStates) |
| ~CMuli3DTexture () |
Constructor & Destructor Documentation
|
Accessible by IBase. The destructor is called when the reference count reaches zero.
|
CMuli3DTexture::CMuli3DTexture |
( |
class CMuli3DDevice * |
i_pParent |
) |
[protected] |
|
|
Accessible by CMuli3DDevice which is the only class that may create a texture. - Parameters:
-
[in] | i_pParent | a pointer to the parent CMuli3DDevice-object. |
|
Member Function Documentation
result CMuli3DTexture::Clear |
( |
uint32 |
i_iMipLevel, |
|
|
const vector4 & |
i_vColor, |
|
|
const m3drect * |
i_pRect |
|
) |
|
|
|
Clears the texture to a given color. - Parameters:
-
[in] | i_iMipLevel | the mip-level to be cleared. |
[in] | i_vColor | color to clear the texture to. |
[in] | i_pRect | rectangle to restrict clearing to. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if the clear-rectangle exceeds the texture's dimensions.
|
|
Accessible by CMuli3DDevice which is the only class that may create a texture. - Parameters:
-
[in] | i_iWidth | width of the texture to be created in pixels. |
[in] | i_iHeight | height of the texture to be created in pixels. |
[in] | i_iMipLevels | number of mip-levels to be created. Specify 0 to create a full mip-chain. |
[in] | i_fmtFormat | format of the texture to be created. Member of the enumeration m3dformat; m3dfmt_r32f, m3dfmt_r32g32f, m3dfmt_r32g32b32f or m3dfmt_r32g32b32a32f. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_outofmemory if memory allocation failed.
e_invalidformat if an invalid format was encountered.
|
|
Sampling this texture requires 2 floating point coordinates.
Implements IMuli3DBaseTexture.
|
|
Returns the format of the texture. Member of the enumeration m3dformat; m3dfmt_r32f, m3dfmt_r32g32f, m3dfmt_r32g32b32f or m3dfmt_r32g32b32a32f.
|
result CMuli3DTexture::GenerateMipSubLevels |
( |
uint32 |
i_iSrcLevel |
) |
|
|
|
Generates mip-sublevels through downsampling (using a box-filter) a given source mip-level. - Parameters:
-
[in] | i_iSrcLevel | the mip-level which will be taken as the starting point. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
|
uint32 CMuli3DTexture::iGetFormatFloats |
( |
|
) |
|
|
|
Returns the number of floats of the format, e [1,4].
|
uint32 CMuli3DTexture::iGetHeight |
( |
uint32 |
i_iMipLevel = 0 |
) |
|
|
|
Returns the height of the given mip-level in pixels. - Parameters:
-
[in] | i_iMipLevel | the mip-level whose height is requested. |
|
uint32 CMuli3DTexture::iGetMipLevels |
( |
|
) |
|
|
|
Returns the number of mip-levels this texture consists of.
|
|
Returns the width of the given mip-level in pixels. - Parameters:
-
[in] | i_iMipLevel | the mip-level whose width is requested. |
|
result CMuli3DTexture::LockRect |
( |
uint32 |
i_iMipLevel, |
|
|
void ** |
o_ppData, |
|
|
const m3drect * |
i_pRect |
|
) |
|
|
|
Returns a pointer to the contents of a given mip-level. - Parameters:
-
[in] | i_iMipLevel | mip-level that is requested, 0 being the largest mip-level. |
[out] | o_ppData | receives the pointer to the texture-data. |
[in] | i_pRect | area that will be locked and accessible. (Pass in 0 to lock entire texture.) |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_invalidstate if the texture is already locked.
e_outofmemory if memory allocation failed.
- Note:
- Locking the entire texture is a lot faster than locking a sub-region, because no lock-buffer has to be created and the application may write to the texture directly.
|
|
Returns a surface-pointer to a given texture mip-level. Calling this function will increase the internal reference count of the surface. Failure to call Release() when finished using the pointer will result in a memory leak. - Parameters:
-
[in] | i_iMipLevel | mip-level, 0 being the largest mip-level. |
|
result CMuli3DTexture::SampleTexture |
( |
vector4 & |
o_vColor, |
|
|
float32 |
i_fU, |
|
|
float32 |
i_fV, |
|
|
float32 |
i_fW, |
|
|
const vector4 * |
i_pXGradient, |
|
|
const vector4 * |
i_pYGradient, |
|
|
const uint32 * |
i_pSamplerStates |
|
) |
[protected, virtual] |
|
|
Accessible by CMuli3DDevice and CMuli3DCubeTexture. (A cube-texture consists of six standard 2d textures and needs access to their sampling function.) Samples the texture and returns the looked-up color. - Parameters:
-
[out] | o_vColor | receives the color of the pixel to be looked up. |
[in] | i_fU | u-component of the lookup-vector. |
[in] | i_fV | v-component of the lookup-vector. |
[in] | i_fW | w-component of the lookup-vector (unused). |
[in] | i_pXGradient | partial derivatives of the texture coordinates with respect to the screen-space x coordinate. If 0 the base mip-level will be chosen and the minification filter will be used for texture sampling. |
[in] | i_pYGradient | partial derivatives of the texture coordinates with respect to the screen-space y coordinate. If 0 the base mip-level will be chosen and the minification filter will be used for texture sampling. |
[in] | i_pSamplerStates | texture sampler states. |
- Returns:
- s_ok if the function succeeds.
Implements IMuli3DBaseTexture.
|
|
Unlocks the given mip-level; modifications to the texture will become active. - Parameters:
-
[in] | i_iMipLevel | mip-level, 0 being the largest mip-level. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
|
|
|