 |
|
|
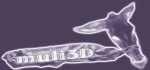 |
Reference: Muli3D: m3dbase.h File Reference
m3dbase.h File Reference
Detailed Description
#include <stdlib.h>
#include <memory.h>
#include <vector>
Go to the source code of this file.
Define Documentation
#define FUNC_FAILED |
( |
res |
|
) |
( res != s_ok ) |
|
|
Macro to test for failure. - Parameters:
-
[in] | res | a function return value. |
- Returns:
- true if the function failed.
|
#define FUNC_FAILING ( void ) |
|
|
Function is only available in debug-builds.
|
#define FUNC_NOTIFY ( void ) |
|
|
Function is only available in debug-builds.
|
#define FUNC_SUCCESSFUL |
( |
res |
|
) |
( res == s_ok ) |
|
|
Macro to test for success. - Parameters:
-
[in] | res | a function return value. |
- Returns:
- true if the function succeeded.
|
#define SAFE_DELETE |
( |
p |
|
) |
{ if( p ) { delete p; p = 0; } } |
|
|
If the pointer is not null, the memory it is pointing to is deleted and the pointer is set to null to ease debugging and to make sure it is not deleted again using SAFE_DELETE(). - Parameters:
-
|
#define SAFE_DELETE_ARRAY |
( |
p |
|
) |
{ if( p ) { delete[] p; p = 0; } } |
|
|
If the pointer is not null, the memory it is pointing to is deleted and the pointer is set to null to ease debugging and to make sure it is not deleted again using SAFE_DELETE_ARRAY(). - Parameters:
-
[in] | p | a pointer to an array. |
|
#define SAFE_RELEASE |
( |
p |
|
) |
{ if( p ) { ( p )->Release(); p = 0; } } |
|
|
If the pointer is not null, the object it is pointing to is released and the pointer is set to null to ease debugging and to make sure it is not released again using SAFE_RELEASE(). - Parameters:
-
[in] | p | a pointer to a reference counted object. |
|
Typedef Documentation
typedef unsigned char byte |
|
typedef signed short int16 |
|
typedef unsigned char uint8 |
|
Enumeration Type Documentation
|
Describes function return values.
- Enumeration values:
-
s_ok |
No errors were encountered, function returned successfully. |
e_unknown |
An unknown error has occured. |
e_invalidparameters |
One or more parameters were invalid. |
e_outofmemory |
Allocation of memory within the function failed. |
e_invalidformat |
A format is invalid for a specific task. |
e_invalidstate |
An invalid state has been found and the function cannot continue. |
|
Function Documentation
|
ftol() performs fast float to integer-conversion. Ensure that fpuTruncate() has been called before using this function, otherwise ftol() will round to the nearest integer. After calling fpuTruncate() it is advised to reset the fpu to rounding-mode using fpuReset(). - Parameters:
-
[in] | f | The floating pointer number to be converted to an integer. |
- Returns:
- an integer.
|
|
|