 |
|
|
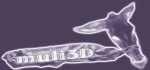 |
Reference: Muli3D: CMuli3DDevice Class Reference
CMuli3DDevice Class Reference#include <m3dcore_device.h>
Inheritance diagram for CMuli3DDevice:
List of all members.
Detailed Description
The Muli3D device.
|
Public Member Functions |
result | CreateCubeTexture (class CMuli3DCubeTexture **o_ppCubeTexture, uint32 i_iEdgeLength, uint32 i_iMipLevels, m3dformat i_fmtFormat) |
result | CreateIndexBuffer (class CMuli3DIndexBuffer **o_ppIndexBuffer, uint32 i_iLength, m3dformat i_fmtFormat) |
result | CreateRenderTarget (class CMuli3DRenderTarget **o_ppVertexFormat) |
result | CreateSurface (class CMuli3DSurface **o_ppSurface, uint32 i_iWidth, uint32 i_iHeight, m3dformat i_fmtFormat) |
result | CreateTexture (class CMuli3DTexture **o_ppTexture, uint32 i_iWidth, uint32 i_iHeight, uint32 i_iMipLevels, m3dformat i_fmtFormat) |
result | CreateVertexBuffer (class CMuli3DVertexBuffer **o_ppVertexBuffer, uint32 i_iLength) |
result | CreateVertexFormat (class CMuli3DVertexFormat **o_ppVertexFormat, const m3dvertexelement *i_pVertexDeclaration, uint32 i_iVertexDeclSize) |
result | CreateVolume (class CMuli3DVolume **o_ppVolume, uint32 i_iWidth, uint32 i_iHeight, uint32 i_iDepth, m3dformat i_fmtFormat) |
result | CreateVolumeTexture (class CMuli3DVolumeTexture **o_ppVolumeTexture, uint32 i_iWidth, uint32 i_iHeight, uint32 i_iDepth, uint32 i_iMipLevels, m3dformat i_fmtFormat) |
result | DrawDynamicPrimitive (uint32 i_iStartVertex, uint32 i_iNumVertices) |
result | DrawIndexedPrimitive (m3dprimitivetype i_PrimitiveType, int32 i_iBaseVertexIndex, uint32 i_iMinIndex, uint32 i_iNumVertices, uint32 i_iStartIndex, uint32 i_iPrimitiveCount) |
result | DrawPrimitive (m3dprimitivetype i_PrimitiveType, uint32 i_iStartVertex, uint32 i_iPrimitiveCount) |
result | GetClippingPlane (m3dclippingplanes i_eIndex, plane &o_plane) |
void | GetDepthBounds (float32 &o_fMinZ, float32 &o_fMaxZ) |
const m3ddeviceparameters & | GetDeviceParameters () |
result | GetRenderState (m3drenderstate i_RenderState, uint32 &o_iValue) |
m3drect | GetScissorRect () |
result | GetTexture (uint32 i_iSamplerNumber, class IMuli3DBaseTexture **o_ppTexture) |
result | GetTextureSamplerState (uint32 i_iSamplerNumber, m3dtexturesamplerstate i_TextureSamplerState, uint32 &o_iState) |
result | GetVertexStream (uint32 i_iStreamNumber, class CMuli3DVertexBuffer **o_ppVertexBuffer, uint32 *o_pOffset, uint32 *o_pStride) |
uint32 | iGetRenderedPixels () |
CMuli3DIndexBuffer * | pGetIndexBuffer () |
CMuli3D * | pGetMuli3D () |
IMuli3DPixelShader * | pGetPixelShader () |
IMuli3DPrimitiveAssembler * | pGetPrimitiveAssembler () |
CMuli3DRenderTarget * | pGetRenderTarget () |
IMuli3DTriangleShader * | pGetTriangleShader () |
CMuli3DVertexFormat * | pGetVertexFormat () |
IMuli3DVertexShader * | pGetVertexShader () |
result | Present (class CMuli3DRenderTarget *i_pRenderTarget) |
result | SampleTexture (vector4 &o_vColor, uint32 i_iSamplerNumber, float32 i_fU, float32 i_fV, float32 i_fW, const vector4 *i_pXGradient, const vector4 *i_pYGradient) |
result | SetClippingPlane (m3dclippingplanes i_eIndex, const plane *i_pPlane) |
result | SetDepthBounds (float32 i_fMinZ, float32 i_fMaxZ) |
result | SetIndexBuffer (class CMuli3DIndexBuffer *i_pIndexBuffer) |
result | SetPixelShader (class IMuli3DPixelShader *i_pPixelShader) |
void | SetPrimitiveAssembler (class IMuli3DPrimitiveAssembler *i_pPrimitiveAssembler) |
result | SetRenderState (m3drenderstate i_RenderState, uint32 i_iValue) |
void | SetRenderTarget (class CMuli3DRenderTarget *i_pRenderTarget) |
result | SetScissorRect (const m3drect &i_ScissorRect) |
result | SetTexture (uint32 i_iSamplerNumber, class IMuli3DBaseTexture *i_pTexture) |
result | SetTextureSamplerState (uint32 i_iSamplerNumber, m3dtexturesamplerstate i_TextureSamplerState, uint32 i_iState) |
void | SetTriangleShader (class IMuli3DTriangleShader *i_pTriangleShader) |
result | SetVertexFormat (class CMuli3DVertexFormat *i_pVertexFormat) |
result | SetVertexShader (class IMuli3DVertexShader *i_pVertexShader) |
result | SetVertexStream (uint32 i_iStreamNumber, class CMuli3DVertexBuffer *i_pVertexBuffer, uint32 i_iOffset, uint32 i_iStride) |
Protected Member Functions |
| CMuli3DDevice (class CMuli3D *i_pParent, const m3ddeviceparameters *i_pDeviceParameters) |
result | Create () |
| ~CMuli3DDevice () |
Constructor & Destructor Documentation
|
Accessible by IBase. The destructor is called when the reference count reaches zero.
|
|
Accessible by CMuli3D which is the only class that may create a device. - Parameters:
-
[in] | i_pParent | a pointer to the parent CMuli3D-object. |
[in] | i_pDeviceParameters | pointer to a m3ddeviceparameters-structure. |
|
Member Function Documentation
result CMuli3DDevice::Create |
( |
|
) |
[protected] |
|
|
Creates and initializes the device. - Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_outofmemory if memory allocation failed.
e_unknown if a presenttarget-specific problem was encountered.
|
|
Creates a cube texture. A pointer to each of the 6 faces can be obtained and used as a target for renderin-operations like a standard 2d texture. - Parameters:
-
[out] | o_ppCubeTexture | receives a pointer to the created texture. |
[in] | i_iEdgeLength | edge length of the texture in pixels. |
[in] | i_iMipLevels | number of miplevels of the new texture; specify 0 to create a full mip-chain. |
[in] | i_fmtFormat | format of the new texture. Member of the enumeration m3dformat; either m3dfmt_index16 or m3dfmt_index32. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_outofmemory if memory allocation failed.
|
|
Creates an index buffer for index storage. - Parameters:
-
[out] | o_ppIndexBuffer | receives a pointer to the created index buffer. |
[in] | i_iLength | length of the index buffer to be created in bytes. |
[in] | i_fmtFormat | format of the index buffer to be created. Member of the enumeration m3dformat; either m3dfmt_index16 or m3dfmt_index32. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_outofmemory if memory allocation failed.
|
|
Creates a render target. - Parameters:
-
[out] | o_ppVertexFormat | receives a pointer to the created render target. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_outofmemory if memory allocation failed.
|
|
Creates a surface. - Parameters:
-
[out] | o_ppSurface | receives a pointer to the created surface. |
[in] | i_iWidth | width of the surface in pixels. |
[in] | i_iHeight | height of the surface in pixels. |
[in] | i_fmtFormat | format of the new surface. Member of the enumeration m3dformat; either m3dfmt_index16 or m3dfmt_index32. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_outofmemory if memory allocation failed.
|
|
Creates a standard 2d texture, which may either be used for texture data storage or as a target for rendering-operations (as frame- or depthbuffer). - Parameters:
-
[out] | o_ppTexture | receives a pointer to the created texture. |
[in] | i_iWidth | width of the texture in pixels. |
[in] | i_iHeight | height of the texture in pixels. |
[in] | i_iMipLevels | number of miplevels of the new texture; specify 0 to create a full mip-chain. |
[in] | i_fmtFormat | format of the new texture. Member of the enumeration m3dformat; either m3dfmt_index16 or m3dfmt_index32. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_outofmemory if memory allocation failed.
|
|
Creates a vertex buffer for vertex storage. - Parameters:
-
[out] | o_ppVertexBuffer | receives a pointer to the created vertex buffer. |
[in] | i_iLength | length of the vertex buffer to be created in bytes. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_outofmemory if memory allocation failed.
|
|
Creates a vertex format from a vertex declaration. A vertex format describes the layout of vertex data in the vertex streams. - Parameters:
-
[out] | o_ppVertexFormat | receives a pointer to the created vertex format. |
[in] | i_pVertexDeclaration | pointer to the vertex declaration. |
[in] | i_iVertexDeclSize | size of the vertex declaration in bytes. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_outofmemory if memory allocation failed.
|
|
Creates a volume. - Parameters:
-
[out] | o_ppVolume | receives a pointer to the created volume. |
[in] | i_iWidth | width of the volume in pixels. |
[in] | i_iHeight | height of the volume in pixels. |
[in] | i_iDepth | depth of the volume in pixels. |
[in] | i_fmtFormat | format of the new surface. Member of the enumeration m3dformat; either m3dfmt_index16 or m3dfmt_index32. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_outofmemory if memory allocation failed.
|
|
Creates a volume texture. Volume texture cannot be used as a target for rendering-operations. - Parameters:
-
[out] | o_ppVolumeTexture | receives a pointer to the created texture. |
[in] | i_iWidth | width of the texture in pixels. |
[in] | i_iHeight | height of the texture in pixels. |
[in] | i_iDepth | depth of the texture in pixels. |
[in] | i_iMipLevels | number of miplevels of the new texture; specify 0 to create a full mip-chain. |
[in] | i_fmtFormat | format of the new texture. Member of the enumeration m3dformat; either m3dfmt_index16 or m3dfmt_index32. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_outofmemory if memory allocation failed.
|
result CMuli3DDevice::DrawDynamicPrimitive |
( |
uint32 |
i_iStartVertex, |
|
|
uint32 |
i_iNumVertices |
|
) |
|
|
|
Renders primitives assembled through the triangle assembler from the currently set vertex streams. - Parameters:
-
[in] | i_iStartVertex | Beginning at this vertex the correct number used for rendering this batch will be read from the vertex streams. |
[in] | i_iNumVertices | specifies the number of vertices that will be used beginning from i_iStartVertex. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_invalidstate if an invalid state was encountered.
|
|
Renders geometric primitives through indexing into an array of vertices. - Parameters:
-
[in] | i_PrimitiveType | member of the enumeration m3dprimitivetype, specifies the primitives' type. |
[in] | i_iBaseVertexIndex | added to each index before accessing a vertex from the array. |
[in] | i_iMinIndex | specifies the minimum index for vertices used during this batch. |
[in] | i_iNumVertices | specifies the number of vertices that will be used beginning from i_iBaseVertexIndex + i_iMinIndex. |
[in] | i_iStartIndex | Location in the index buffer to start reading from. |
[in] | i_iPrimitiveCount | Amount of primitives to render. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_invalidstate if an invalid state was encountered.
|
|
Renders nonindexed primitives of the specified type from the currently set vertex streams. - Parameters:
-
[in] | i_PrimitiveType | member of the enumeration m3dprimitivetype, specifies the primitives' type. |
[in] | i_iStartVertex | Beginning at this vertex the correct number used for rendering this batch will be read from the vertex streams. |
[in] | i_iPrimitiveCount | Amount of primitives to render. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_invalidstate if an invalid state was encountered.
|
|
- Parameters:
-
[in] | i_eIndex | clipping plane index (member of the enumeration m3dclippingplanes) starting from m3dcp_user0. |
[out] | o_plane | reference to the output plane. |
- Returns:
- e_invalidparameters if the index is invalid.
e_invalidstate if the specified clipping has not been set.
|
void CMuli3DDevice::GetDepthBounds |
( |
float32 & |
o_fMinZ, |
|
|
float32 & |
o_fMaxZ |
|
) |
|
|
|
Returns the currently set depth bounding values.
|
|
Returns the device parameters.
|
|
Retrieves the value of a renderstate. - Parameters:
-
[in] | i_RenderState | member of the enumeration m3drenderstate. |
[out] | o_iValue | receives the value of the renderstate. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
|
m3drect CMuli3DDevice::GetScissorRect |
( |
|
) |
|
|
|
Returns the currently set scissor rect.
|
|
Returns a pointer to the active texture of a given sampler. Calling this function will increase the internal reference count of the texture. Failure to call Release() when finished using the pointer will result in a memory leak. - Parameters:
-
[in] | i_iSamplerNumber | number of the sampler. |
[out] | o_ppTexture | receives a pointer to the texture. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
|
|
Retrieves a sampler state. - Parameters:
-
[in] | i_iSamplerNumber | number of the sampler. |
[in] | i_TextureSamplerState | sampler state. Member of the enumeration m3dtexturesamplerstate. |
[out] | o_iState | receives the value of the sampler state. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
|
|
Returns a pointer to the active vertex buffer of a given stream. Calling this function will increase the internal reference count of the vertex buffer. Failure to call Release() when finished using the pointer will result in a memory leak. - Parameters:
-
[in] | i_iStreamNumber | number of the stream. |
[out] | o_ppVertexBuffer | receives a pointer to the vertex buffer. |
[out] | o_pOffset | receives the offset from the start of the vertex buffer in bytes. (In case this value doesn't need to be retrieved, pass 0 as parameter.) |
[out] | o_pStride | receives the stride in bytes. (In case this value doesn't need to be retrieved, pass 0 as parameter.) |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
|
uint32 CMuli3DDevice::iGetRenderedPixels |
( |
|
) |
|
|
|
Returns the number of pixels that passed the depth-test during the last Draw*Primitive() call.
|
|
Returns a pointer to the active index buffer. Calling this function will increase the internal reference count of the index buffer. Failure to call Release() when finished using the pointer will result in a memory leak.
|
CMuli3D * CMuli3DDevice::pGetMuli3D |
( |
|
) |
|
|
|
Returns a pointer to the Muli3D instance. Calling this function will increase the internal reference count of the Muli3D instance. Failure to call Release() when finished using the pointer will result in a memory leak.
|
|
Returns a pointer to the active pixel shader. Calling this function will increase the internal reference count of the pixel shader. Failure to call Release() when finished using the pointer will result in a memory leak.
|
|
Returns a pointer to the active triangle assembler. Calling this function will increase the internal reference count of the primitive assembler. Failure to call Release() when finished using the pointer will result in a memory leak.
|
|
Returns a pointer to the active render target. Calling this function will increase the internal reference count of the render target. Failure to call Release() when finished using the pointer will result in a memory leak.
|
|
Returns a pointer to the active triangle shader. Calling this function will increase the internal reference count of the triangle shader. Failure to call Release() when finished using the pointer will result in a memory leak.
|
|
Returns a pointer to the active vertex format. Calling this function will increase the internal reference count of the vertex format. Failure to call Release() when finished using the pointer will result in a memory leak.
|
|
Returns a pointer to the active vertex shader. Calling this function will increase the internal reference count of the vertex shader. Failure to call Release() when finished using the pointer will result in a memory leak.
|
|
Presents the contents of a given rendertarget's colorbuffer. - Parameters:
-
[in] | i_pRenderTarget | the rendertarget to be presented. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
e_invalidformat if an invalid format was encountered.
e_invalidstate if an invalid state was encountered.
e_unknown if a present-target related problem was encountered.
|
result CMuli3DDevice::SampleTexture |
( |
vector4 & |
o_vColor, |
|
|
uint32 |
i_iSamplerNumber, |
|
|
float32 |
i_fU, |
|
|
float32 |
i_fV, |
|
|
float32 |
i_fW, |
|
|
const vector4 * |
i_pXGradient, |
|
|
const vector4 * |
i_pYGradient |
|
) |
|
|
|
Samples the texture and returns the looked-up color. - Parameters:
-
[out] | o_vColor | receives the color of the pixel to be looked up. |
[in] | i_iSamplerNumber | number of the sampler. |
[in] | i_fU | u-component of the lookup-vector. |
[in] | i_fV | v-component of the lookup-vector. |
[in] | i_fW | w-component of the lookup-vector. |
[in] | i_pXGradient | partial derivatives of the texture coordinates with respect to the screen-space x coordinate. If 0 the base mip-level will be chosen and the minification filter will be used for texture sampling. |
[in] | i_pYGradient | partial derivatives of the texture coordinates with respect to the screen-space y coordinate. If 0 the base mip-level will be chosen and the minification filter will be used for texture sampling. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
|
|
Sets an user-specified clipping plane. - Parameters:
-
[in] | i_eIndex | clipping plane index (member of the enumeration m3dclippingplanes) starting from m3dcp_user0. |
[in] | i_pPlane | pointer to clipping plane. Pass 0 to disable a clipping plane. |
- Returns:
- e_invalidparameters if the index is invalid.
|
|
Sets the bounding values for depth. - Parameters:
-
[in] | i_fMinZ | minimum allowed depth value, e [0;1], default: 0. |
[in] | i_fMaxZ | maximum allowed depth value, e [0;1], default: 1. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if the bound values are invalid.
|
|
Sets the index buffer. - Parameters:
-
[in] | i_pIndexBuffer | pointer to the index buffer. |
- Returns:
- s_ok if the function succeeds.
|
|
Sets the pixel shader. - Parameters:
-
[in] | i_pPixelShader | pointer to the pixel shader. |
- Returns:
- s_ok if the function succeeds.
e_invalidstate if the type of pixelshader is invalid.
|
|
Sets the triangle assembler. - Parameters:
-
[in] | i_pPrimitiveAssembler | pointer to the primitive assembler. |
|
|
Sets a renderstate. - Parameters:
-
[in] | i_RenderState | member of the enumeration m3drenderstate. |
[in] | i_iValue | value to be set. Some renderstates require floating point values, which can be set through casting to a uint32-pointer and dereferencing it. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
|
|
Sets the render target. - Parameters:
-
[in] | i_pRenderTarget | pointer to the render target. |
|
result CMuli3DDevice::SetScissorRect |
( |
const m3drect & |
i_ScissorRect |
) |
|
|
|
Sets the scissor rect. - Parameters:
-
[in] | i_ScissorRect | the scissor rect. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if the scissor rectangle is invalid.
|
|
Sets a vertex buffer to a given sampler. - Parameters:
-
[in] | i_iSamplerNumber | number of the sampler. |
[in] | i_pTexture | pointer to the texture. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
|
|
Sets a sampler state. - Parameters:
-
[in] | i_iSamplerNumber | number of the sampler. |
[in] | i_TextureSamplerState | sampler state. Member of the enumeration m3dtexturesamplerstate. |
[in] | i_iState | value to be set. Some sampler states require floating point values, which can be set through casting to a uint32-pointer and dereferencing it. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
|
|
Sets the triangle shader. - Parameters:
-
[in] | i_pTriangleShader | pointer to the triangle shader. |
|
|
Sets the vertex format. - Parameters:
-
[in] | i_pVertexFormat | pointer to the vertex format. |
- Returns:
- s_ok if the function succeeds.
|
|
Sets the vertex shader. - Parameters:
-
[in] | i_pVertexShader | pointer to the vertex shader. |
- Returns:
- s_ok if the function succeeds.
e_invalidstate if the type of a vertex shader output register is invalid.
|
|
Sets a vertex buffer to a given stream. - Parameters:
-
[in] | i_iStreamNumber | number of the stream. |
[in] | i_pVertexBuffer | pointer to the vertex buffer. |
[in] | i_iOffset | offset from the start of the vertex buffer in bytes. |
[in] | i_iStride | stride in bytes. |
- Returns:
- s_ok if the function succeeds.
e_invalidparameters if one or more parameters were invalid.
|
|
|